Presently we are using many Templated we b control in asp.net as GridView, Detailview etc. In Templeted Control developer is free to change the appearance of control according to their reqiurment. So basically a Templeted Control provide sepration between data and presentation. Similarly we can also design Templeted Web User Control. So the appearance of control will depend on developer. The step creating template webn user control is given below.
Like user controls, the templated user control is only reusable in the same Web site.
The templated user control must provide a container class that is a naming container and has properties that are accessible to the host page. The template contains the user interface for the templated user control and is supplied by the page developer at design time. A template can contain controls and markup.
Here I am creating a templated web user control for User Address
1. In Visual studio open a web project it will contain a default.aspx by default. Add a web user control and place an ASP.NET Placeholder control where you want the template to appear.
2.(UserAddress.cs) Add a new class to the App_Code folder in your Web site that contains the template's naming container class. This class inherits from Control, implements the INamingContainer, and contains a public property for each data element that is visible to the template. The container control contains an instance of the template when it is rendered.
3. In the user control's code-behind page, add public properties to add data to Templates’naming contailer.Implement a public property of type Itemplate and ITemplate.Apply the TemplateContainerAttribute to the ITemplate property and pass the type of the template's( Eg. UserAddress) naming container class as the argument to the constructor of the attribute. Also, apply the PersistenceModeAttribute to the ITemplate property and pass the enumeration value of PersistenceMode.InnerProperty into its constructor.
4.(TempletedUserControl.ascx.cs) In the Page_Init method of the user control, test for the ITemplate property being set. If the ITemplate property is set, create an instance of the naming container class and create an instance of the template in the naming container. Add the naming container instance to the Controls property of the PlaceHolder server control.In Page_Load method call Databind method.
6. In Default.aspx page set the properies to read data and define the template for data presentation.
Sample code for creating Templated Web User control is given below.
UserAddress.cs
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
/// <summary>
/// Summary description for UserAddress
/// </summary>
public class UserAddress:Control,INamingContainer
{
public UserAddress(string name,string address,string city , string state,string country,int zip)
{
Name = name;
Address = address;
City = city;
State = state;
Country = country;
Zip = zip;
}
public string Address
{
get
{
return _address;
}
set
{
_address = value;
}
}
string _address;
public string Name
{
get
{ return _name; }
set
{
_name = value;
}
}
string _name;
public string State
{
get
{
return _state;
}
set
{
_state = value;
}
}
string _state;
public string City
{
get
{
return _City;
}
set
{
_City = value;
}
}
string _City;
public string Country
{
get
{
return _Country;
}
set
{
_Country = value;
}
}
string _Country;
public int Zip
{
get
{
return _zip;
}
set
{
_zip = value;
}
}
int _zip;
}
TemplatedUserControl.ascx
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="TepletedUserControl.ascx.cs" Inherits="TepletedUserControl" %>
<asp:PlaceHolder ID="PlaceHolder1" runat="server"></asp:PlaceHolder>
TemplatedUserControl.ascx.cs
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
public partial class TepletedUserControl : System.Web.UI.UserControl
{
ITemplate userAddress;
[TemplateContainer(typeof(UserAddress))]
[PersistenceMode(PersistenceMode.InnerProperty)]
public ITemplate UserAddressTempete
{
get
{
return userAddress;
}
set
{
userAddress = value;
}
}
public string Address
{
get
{
return _address;
}
set
{
_address = value;
}
}
string _address;
public string Name
{
get
{ return _name; }
set
{
_name = value;
}
}
string _name;
public string State
{
get
{
return _state;
}
set
{
_state = value;
}
}
string _state;
public string City
{
get
{
return _City;
}
set
{
_City = value;
}
}
string _City;
public string Country
{
get
{
return _Country;
}
set
{
_Country = value;
}
}
string _Country;
public int Zip
{
get
{
return _zip;
}
set
{
_zip = value;
}
}
int _zip;
protected void Page_Load(object sender, EventArgs e)
{
DataBind();
}
protected void Page_Init(object sender, EventArgs e)
{
PlaceHolder1.Controls.Clear();
if (UserAddressTempete == null)
{
PlaceHolder1.Controls.Add(new LiteralControl("No Template is defined"));
return;
}
UserAddress uadd = new UserAddress(Name, Address, City, State, Country, Zip);
UserAddressTempete.InstantiateIn(uadd);
PlaceHolder1.Controls.Add(uadd);
}
}
Default.aspx.cs
there is no need to write any thing regarding thisin this file.
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<%@ Register Src="TepletedUserControl.ascx" TagName="TepletedUserControl" TagPrefix="uc1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<uc1:TepletedUserControl id="TepletedUserControl1" runat="server" Name="Som Nath" Address="CST " City="Mumbai" State="Maharashtra" Country="India" Zip="400079">
<UserAddressTempete>
<h1> User Address</h1>
<div style="background-color:Red"><h2> Name: </h2>
<%#Container.Name %>
</div>
<div style="background-color:Green"><h2>Address:</h2>
<%#Container.Address %></div>
<div style="background-color:Green " ><h2> City: </h2>
<%#Container.City%>
</div>
<div style="background-color:Red"><h2>State:</h2>
<%#Container.State%>
</div>
<div style="background-color:Green"><h2>Country:</h2>
<%#Container.Country%>
</div>
<div style="background-color:Red"><h2>Zip:</h2>
<%#Container.Zip%>
</div>
</UserAddressTempete>
</uc1:TepletedUserControl> </div>
</form>
</body>
</html>
See
Tuesday, November 27, 2007
USER control in Asp.net 2.0
User Control:
As the name suggest user control are made by developer based on there requirement which are not available. In single word you can say user control is customized control based on user requirement.
Many time webpage contains similar controls eg. Address it may be billing address or shipping address, or there are many other case of using similar controls. You can create a user control containing the name, address, city, state, and zip code and drop it onto a Web page where needed . It will save time and provide consistency in form of appearance.
There are two way for making User control
1. Using web userControl.ascx
2. Converting A standard Asp.net web page into User control page.
You can create a user control in Visual Studio 2005 by choosing Website, Add New Item, Web User Control
<%@ Control Language="C#" AutoEventWireup="true"
CodeFile="MyControl.ascx.cs" Inherits="MyControl" %>
It has .ascx extension making sure that it cannot executed standalone. I mean you have to use .aspx page to use the user control. All other control will same as on .aspx page.
Converting .aspx page to uaser control
Remove the , , and
As the name suggest user control are made by developer based on there requirement which are not available. In single word you can say user control is customized control based on user requirement.
Many time webpage contains similar controls eg. Address it may be billing address or shipping address, or there are many other case of using similar controls. You can create a user control containing the name, address, city, state, and zip code and drop it onto a Web page where needed . It will save time and provide consistency in form of appearance.
There are two way for making User control
1. Using web userControl.ascx
2. Converting A standard Asp.net web page into User control page.
You can create a user control in Visual Studio 2005 by choosing Website, Add New Item, Web User Control
<%@ Control Language="C#" AutoEventWireup="true"
CodeFile="MyControl.ascx.cs" Inherits="MyControl" %>
It has .ascx extension making sure that it cannot executed standalone. I mean you have to use .aspx page to use the user control. All other control will same as on .aspx page.
Converting .aspx page to uaser control
Remove the , , and
Tuesday, November 20, 2007
GENERAL PACKET RADIO SERVICE (GPRS)
The standard bandwidth of 9.6kbps available for the data services is not sufficient for the requirements of today’s computers .To enhance the data transmission capabilities of GSM , two basic approaches were developed…….
1.High speed circuit switched data(HSCSD):---------
The first one is high speed circuit switched data . It allocates several TDMA slots within a TDMA frame .This allocation can be asymmetrical ,i.e., more slots can be allocated on the downlink than on the uplink ,which fits the typical user behavior of downloading more data .One problem of this configuration is that the MS is required to send and receive at the same time .It also has some disadvantages as it uses the connection oriented mechanisms of GSM . These are not at all efficient for computer data traffic, which is typically bursty and asymmetrical.
For n channels , HSCSD requires n times signaling during handover, connection set up and release . Each channel is treated separately. The probability of blocking or services degradation increase during handover , as in this case a BSC has to check resources for n channels , not just one .
2. GENERAL PACKET RADIO SERVICE (GPRS) :----------
The next step towards more flexible and powerful data transmission is GPRS .It is more efficient and cheapest because in this mode charging is based on volume not on connection time like HSCSD . The main advantage of GPRS is the ‘always on’ characteristic no connection has to be setup prior to data transfer. In GPRS time slots are not allocated in a fixed , pre determined manner but on demand .All time slots can be shared by the active users ;up-down link are allocated separately. Allocation of the slots is based on current load and operator preferences . The GPRS concept is independent of channel characteristic and of the type of channel ,and does not limit the maximum data rate.
GPRS offers a point-to-point (PTP) packet transfer service. One of the PTP version offered is the PTP connection oriented network services (PTP-CONS), which includes the ability of GPRS to maintain a virtual circuit upon change of the cell within the GSM network. The other PTP version offered is the PTP connectionless network service (PTP-CLNS) , which support applications that based on the internet protocol IP. Multicasting , called point-to-multipoint(PTM) service. Users of GPRS can specify a QoS-profile. This determine the service precedence ,reliability class and delay class of the transmission , and user data throughput. GPRS exhibit a large jitter compared to fixed networks.
The GPRS architecture introduces two new network elements , which are called GPRS support nodes(GSN) and work like router .The gateway GPRS node (GGSN) is the interworking unit between the GPRS network and packet data networks(PDN). This node contains routing information for GPRS users ,performs address conversions , and tunnels data to a user via encapsulation . The GGSN is connected to external networks to external networks via Gi interface and transfer packets to the SGSN via an IP-based GPRS backbone network. The other new element is the serving GPRS supports node(SGSN) which supports the MS via the Gb interface. Packet data is transmitted from a PDN , via the GGSN and SGSN directly to the BSS and finally to the MS . The MSC which is responsible for data transport in the traditional circuit-switched GSM, is used for signaling in the GPRS scenario. Before sending any data over the GPRS network ,an MS must attach to it ,following the procedure of the mobility management .The attachment procedure includes assign a temporal identifier ,called a temporary logical link identity (TLLI) ,and a ciphering key sequence number(CKSN) for data encryption.
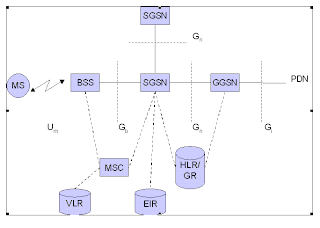
GPRS ARCHITECTURE REFERENCE MODEL
The protocol architecture of the transmission for GPRS is given in the figure . All the data within the GPRS backbone ,i.e. ,between the GSNs , is transferred using the GPRS tunneling protocol(GTP) . GTP can use two different transport layer protocols , TCP or UDP. The network protocol for the GPRS backbone is IP. To adapt the different characteristics of the underlying networks , the subnetwork dependent convergence protocol(SNDCP) is used between an SGSN and the MS. A base station subsystem GPRS protocol(BSSGP) is used to convey routing and QoS-related information between the BSS and SGSN.BSSGP does not perform error connection and works on the top of a frame relay network .Finally , radio link dependent protocol are needed to transfer data over the Um interface .
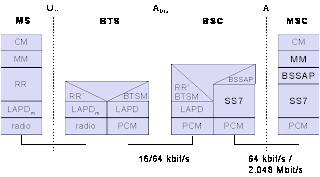
GPRS TRANSMISSION PLANE PROTOCOL REFEERNCE MODEL
The radio link protocol (RLC) provides a reliable link , while the MAC control access with signaling procedure for the radio channel and mapping of LLC frames onto the GSM physical channels . Capacity can be allocated on demand and shared between circuit-switched channels and GPRS. This allocation can be done dynamically with load supervision or alternatively, capacity can be pre-allocated. The application uses TCP on top of IP , IP packets are tunneled to the GGSN, witch forwards them into PDN . All PDNs forward their packets for a GPRS user to the GGSN ,the GGSN asks the current SGSN for tunnel parameters ,and forward their packets via SGSN to the MS. All MS are assigned private IP address which are then translated into global address at GGSN using NAT. The advantage of this approach is the inherent protection of MSs from attacks. Private address are not routed through the internet so it not possible to reach an MS from the internet. This is also a disadvantage if MS wants to offer a service using a fixed , globally visible IP address.
1.High speed circuit switched data(HSCSD):---------
The first one is high speed circuit switched data . It allocates several TDMA slots within a TDMA frame .This allocation can be asymmetrical ,i.e., more slots can be allocated on the downlink than on the uplink ,which fits the typical user behavior of downloading more data .One problem of this configuration is that the MS is required to send and receive at the same time .It also has some disadvantages as it uses the connection oriented mechanisms of GSM . These are not at all efficient for computer data traffic, which is typically bursty and asymmetrical.
For n channels , HSCSD requires n times signaling during handover, connection set up and release . Each channel is treated separately. The probability of blocking or services degradation increase during handover , as in this case a BSC has to check resources for n channels , not just one .
2. GENERAL PACKET RADIO SERVICE (GPRS) :----------
The next step towards more flexible and powerful data transmission is GPRS .It is more efficient and cheapest because in this mode charging is based on volume not on connection time like HSCSD . The main advantage of GPRS is the ‘always on’ characteristic no connection has to be setup prior to data transfer. In GPRS time slots are not allocated in a fixed , pre determined manner but on demand .All time slots can be shared by the active users ;up-down link are allocated separately. Allocation of the slots is based on current load and operator preferences . The GPRS concept is independent of channel characteristic and of the type of channel ,and does not limit the maximum data rate.
GPRS offers a point-to-point (PTP) packet transfer service. One of the PTP version offered is the PTP connection oriented network services (PTP-CONS), which includes the ability of GPRS to maintain a virtual circuit upon change of the cell within the GSM network. The other PTP version offered is the PTP connectionless network service (PTP-CLNS) , which support applications that based on the internet protocol IP. Multicasting , called point-to-multipoint(PTM) service. Users of GPRS can specify a QoS-profile. This determine the service precedence ,reliability class and delay class of the transmission , and user data throughput. GPRS exhibit a large jitter compared to fixed networks.
The GPRS architecture introduces two new network elements , which are called GPRS support nodes(GSN) and work like router .The gateway GPRS node (GGSN) is the interworking unit between the GPRS network and packet data networks(PDN). This node contains routing information for GPRS users ,performs address conversions , and tunnels data to a user via encapsulation . The GGSN is connected to external networks to external networks via Gi interface and transfer packets to the SGSN via an IP-based GPRS backbone network. The other new element is the serving GPRS supports node(SGSN) which supports the MS via the Gb interface. Packet data is transmitted from a PDN , via the GGSN and SGSN directly to the BSS and finally to the MS . The MSC which is responsible for data transport in the traditional circuit-switched GSM, is used for signaling in the GPRS scenario. Before sending any data over the GPRS network ,an MS must attach to it ,following the procedure of the mobility management .The attachment procedure includes assign a temporal identifier ,called a temporary logical link identity (TLLI) ,and a ciphering key sequence number(CKSN) for data encryption.
GPRS ARCHITECTURE REFERENCE MODEL
The protocol architecture of the transmission for GPRS is given in the figure . All the data within the GPRS backbone ,i.e. ,between the GSNs , is transferred using the GPRS tunneling protocol(GTP) . GTP can use two different transport layer protocols , TCP or UDP. The network protocol for the GPRS backbone is IP. To adapt the different characteristics of the underlying networks , the subnetwork dependent convergence protocol(SNDCP) is used between an SGSN and the MS. A base station subsystem GPRS protocol(BSSGP) is used to convey routing and QoS-related information between the BSS and SGSN.BSSGP does not perform error connection and works on the top of a frame relay network .Finally , radio link dependent protocol are needed to transfer data over the Um interface .
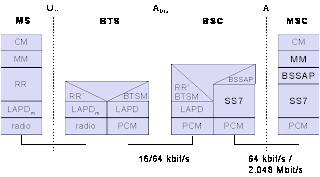
GPRS TRANSMISSION PLANE PROTOCOL REFEERNCE MODEL
The radio link protocol (RLC) provides a reliable link , while the MAC control access with signaling procedure for the radio channel and mapping of LLC frames onto the GSM physical channels . Capacity can be allocated on demand and shared between circuit-switched channels and GPRS. This allocation can be done dynamically with load supervision or alternatively, capacity can be pre-allocated. The application uses TCP on top of IP , IP packets are tunneled to the GGSN, witch forwards them into PDN . All PDNs forward their packets for a GPRS user to the GGSN ,the GGSN asks the current SGSN for tunnel parameters ,and forward their packets via SGSN to the MS. All MS are assigned private IP address which are then translated into global address at GGSN using NAT. The advantage of this approach is the inherent protection of MSs from attacks. Private address are not routed through the internet so it not possible to reach an MS from the internet. This is also a disadvantage if MS wants to offer a service using a fixed , globally visible IP address.
HandOver In GSM
Cellular system required handover procedure as single cells do not cover the whole service area. There are two basic reason for a handover ………
1. The station moves out of the range of BTS or a certain antenna of a BTS respectively .The received signal level decreases continuously until it falls below the minimal requirements for communication .The error rate may increase due to interface , the distance to the BTS may be too high etc. All these effect may diminish the quality of the radio link and make radio transmission impossible in near feature .
2. The wired infrastructure may decide that the traffic in one cell is too high and shift some MS to other cells with a lower load .Handover may be due to load balancing .
The handover are four type
1. Intra-cell handover
2. Inter-cell , Intra-BSC handover
3. Inter-BDC , intra-MSC handover
4. Inter-MSC handover
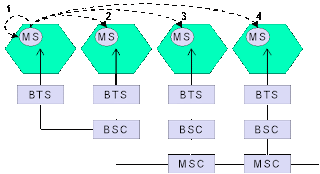
TYPES OF HANDOVER IN GSM SYSTEM
To provide all necessary information for a handover due to weak link , MS and BTS both perform periodic measurement of the downlink and uplink signal quality respectively. Measurement report are sent by the MS about every half-second and contain the quality of the current link used for information as well as the quality of certain channels in neighboring cells(BCCH). Handover is of two type Soft handover and hard handover. In hard handover MS can only talk with a single BTS at a time when changing from one cell to other . But in soft handover MS can talk old and new BTS simultaneously .Soft handover is used in CDMA technology while Hard handover is used in GSM technology. The procedure for handover is given below.
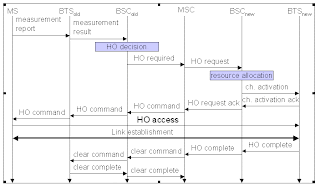
INTRA-MSC HANDOVER
1. The station moves out of the range of BTS or a certain antenna of a BTS respectively .The received signal level decreases continuously until it falls below the minimal requirements for communication .The error rate may increase due to interface , the distance to the BTS may be too high etc. All these effect may diminish the quality of the radio link and make radio transmission impossible in near feature .
2. The wired infrastructure may decide that the traffic in one cell is too high and shift some MS to other cells with a lower load .Handover may be due to load balancing .
The handover are four type
1. Intra-cell handover
2. Inter-cell , Intra-BSC handover
3. Inter-BDC , intra-MSC handover
4. Inter-MSC handover
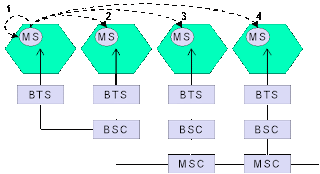
TYPES OF HANDOVER IN GSM SYSTEM
To provide all necessary information for a handover due to weak link , MS and BTS both perform periodic measurement of the downlink and uplink signal quality respectively. Measurement report are sent by the MS about every half-second and contain the quality of the current link used for information as well as the quality of certain channels in neighboring cells(BCCH). Handover is of two type Soft handover and hard handover. In hard handover MS can only talk with a single BTS at a time when changing from one cell to other . But in soft handover MS can talk old and new BTS simultaneously .Soft handover is used in CDMA technology while Hard handover is used in GSM technology. The procedure for handover is given below.
INTRA-MSC HANDOVER
Security in GSM
GSM offers several security services using confidential information stored in the AuC and in the individual SIM.The SIM stores personal,secret data and is protected with a PIN.The security services offered by the GSM are explained below:---
¨Access control and authentication: The first step indicates authentication of a valid user for the SIM.The user needs a secret PIN to access the SIM.
¨Confidentiality: ----
All users related data is encrypted.after authentication, BTS and MS apply encryption to voice, data, and signaling. This exists between MS and BTS.
¨Anonymity: ---
To provide user anonymity, all data is encrypted before transmission, and user identifiers are not used over the air. Instead GSM transmits a temporary identifier (TMSI), which is newly assigned by the VLR after each location update.
Three algorithms have been assigned to provide security services in GSM
Algorithm3 is used for authentication, A5 for encryption, and A8 for the generation of the cipher key.
AUTHENTICATION:--------
Authentication is based on the SIM ,which stores the individual authentication key Ki,
The user identification IMSI ,and the algorithm used for authentication A3. Authentication uses a challenge-response method : the access control generate a random number RAND as challenge , and the SIM within the MS answer with SRES as response. The AuC performs basic generation of random values RAND , signed responses SRES , and cipher keys Kc for each IMSI and then forwards information to the HLR ,the current VLR request the appropriate values for RANSD ,SRES ,and Kc from the HLR. For authentication the VLR sends the random value RAND to the SIM . Both sides , network and subscriber module , perform the same operation with RAND and the key Ki, called A3. The MS sends back the SRES generated by the SIM ; the VLR can now compare both values .If they are the same ,the VLR accepts the subscriber, otherwise the subscriber is rejected.
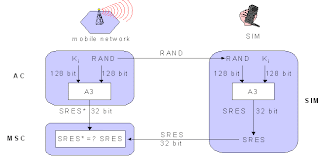
ENCRYPTION :--
To maintain privacy , all message containing user related information are encrypted in GSM over all interface . After authentication ,MS and BSS can start using encryption by applying the cipher key Kc. Kc is generated using the individual key Ki and a random value by applying algorithm A8. The key Kc is not transmitted over the air interface. MS and BTS can now encrypt and decrypt data using the algorithm A5 and cipher key Kc.
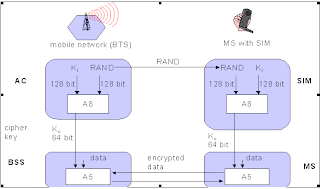
DATA ENCRYPTION
¨Access control and authentication: The first step indicates authentication of a valid user for the SIM.The user needs a secret PIN to access the SIM.
¨Confidentiality: ----
All users related data is encrypted.after authentication, BTS and MS apply encryption to voice, data, and signaling. This exists between MS and BTS.
¨Anonymity: ---
To provide user anonymity, all data is encrypted before transmission, and user identifiers are not used over the air. Instead GSM transmits a temporary identifier (TMSI), which is newly assigned by the VLR after each location update.
Three algorithms have been assigned to provide security services in GSM
Algorithm3 is used for authentication, A5 for encryption, and A8 for the generation of the cipher key.
AUTHENTICATION:--------
Authentication is based on the SIM ,which stores the individual authentication key Ki,
The user identification IMSI ,and the algorithm used for authentication A3. Authentication uses a challenge-response method : the access control generate a random number RAND as challenge , and the SIM within the MS answer with SRES as response. The AuC performs basic generation of random values RAND , signed responses SRES , and cipher keys Kc for each IMSI and then forwards information to the HLR ,the current VLR request the appropriate values for RANSD ,SRES ,and Kc from the HLR. For authentication the VLR sends the random value RAND to the SIM . Both sides , network and subscriber module , perform the same operation with RAND and the key Ki, called A3. The MS sends back the SRES generated by the SIM ; the VLR can now compare both values .If they are the same ,the VLR accepts the subscriber, otherwise the subscriber is rejected.
ENCRYPTION :--
To maintain privacy , all message containing user related information are encrypted in GSM over all interface . After authentication ,MS and BSS can start using encryption by applying the cipher key Kc. Kc is generated using the individual key Ki and a random value by applying algorithm A8. The key Kc is not transmitted over the air interface. MS and BTS can now encrypt and decrypt data using the algorithm A5 and cipher key Kc.
DATA ENCRYPTION
LOCALIZATION AND CALLING
One fundamental feature of GSM system is the automatic , worldwide localization of users. GSM perform periodic location updates even if a user does not use the MS.The HLR always contains information about the current location , and VLR currently responsible for the MS informs the HLR about location changes. As soon as an MS moves into the range of a new VLR , the HLR sends all user data needed to new VLR.
Changing position of services is also called roaming.
To locate an MS and to address the MS following number are required………..
Mobile station inter national ISDN number (MSISDN) :---------
The only important number for a user of GSM in is the phone number. Phone no is associated with SIM ,which is personalized for a user . This no consists of country code (CC) ,the national destination code(NDC) , and subscriber number (SN) .
International mobile subscriber identity(IMSI) :-------
GSM uses the IMSI for internal unique identification of a subscriber .it consists of mobile country code (MCC) ,the mobile network code(MNC) , and mobile subscriber identification identity(MSIN).
Temporary mobile subscriber identity(TMSI):
To hide the IMSI ,which would give the exact identity of the user signaling over the air interface ,GSM uses the 4 byte TMSI for local subscriber identification .TMSI is selected by the current VLR and is only valid temporarily and within the location area of VLR.
Mobile station roaming number(MSRN) :---Another temporary address that hides the location of a subscriber is MSRN. MSRN contains the current visitor country code(VCC) ,the visitor national destination code (VNDC)
The steps involve in calling from a fixed network to a MS (MTC) , and from a MS to fixed network(MOC) is given below…..
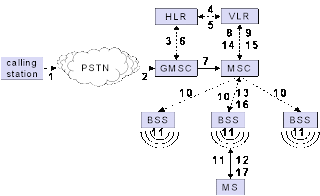
MOBILE TERMINATED CALL(MTC)
1: calling a GSM subscriber
2: forwarding call to GMSC
3: signal call setup to HLR
4, 5: request MSRN from VLR
6: forward responsible
MSC to GMSC
7: forward call to
current MSC
8, 9: get current status of MS
10, 11: paging of MS
12, 13: MS answers
14, 15: security checks
16, 17: set up connection
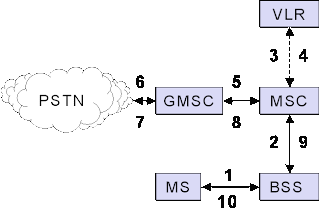
MOBILE OREGINATED CALL (MOC)
1, 2: connection request
3, 4: security check
5-8: check resources (free circuit)
9-10: set up call
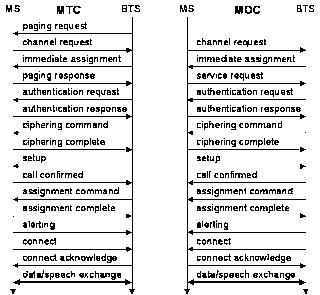
MESSAGE FLOW FOR MTC AND MOC
Changing position of services is also called roaming.
To locate an MS and to address the MS following number are required………..
Mobile station inter national ISDN number (MSISDN) :---------
The only important number for a user of GSM in is the phone number. Phone no is associated with SIM ,which is personalized for a user . This no consists of country code (CC) ,the national destination code(NDC) , and subscriber number (SN) .
International mobile subscriber identity(IMSI) :-------
GSM uses the IMSI for internal unique identification of a subscriber .it consists of mobile country code (MCC) ,the mobile network code(MNC) , and mobile subscriber identification identity(MSIN).
Temporary mobile subscriber identity(TMSI):
To hide the IMSI ,which would give the exact identity of the user signaling over the air interface ,GSM uses the 4 byte TMSI for local subscriber identification .TMSI is selected by the current VLR and is only valid temporarily and within the location area of VLR.
Mobile station roaming number(MSRN) :---Another temporary address that hides the location of a subscriber is MSRN. MSRN contains the current visitor country code(VCC) ,the visitor national destination code (VNDC)
The steps involve in calling from a fixed network to a MS (MTC) , and from a MS to fixed network(MOC) is given below…..
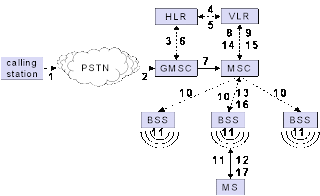
MOBILE TERMINATED CALL(MTC)
1: calling a GSM subscriber
2: forwarding call to GMSC
3: signal call setup to HLR
4, 5: request MSRN from VLR
6: forward responsible
MSC to GMSC
7: forward call to
current MSC
8, 9: get current status of MS
10, 11: paging of MS
12, 13: MS answers
14, 15: security checks
16, 17: set up connection
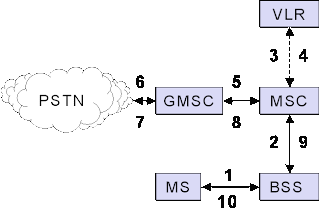
MOBILE OREGINATED CALL (MOC)
1, 2: connection request
3, 4: security check
5-8: check resources (free circuit)
9-10: set up call
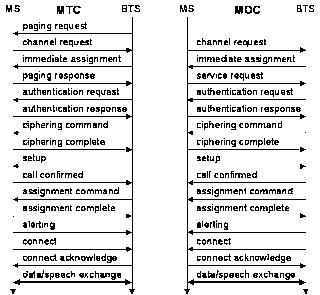
MESSAGE FLOW FOR MTC AND MOC
PROTOCOLS
The protocol system architecture of GSM network is given below. The Um interface is the most important interface. Layer 1,the physical , handles all radio specific functions. This includes the creation of bursts according to the five different formats , multiplexing of bursts into a TDMA frame , synchronization with the BTS , detection of idle channels ,and measurement of the channel quality on the downlink . The physical layer at Um uses GMSK for digital modulation and perform encryption/decryption of data i.e. encryption is not performed end to end but only between MS and BSS over the air interface.
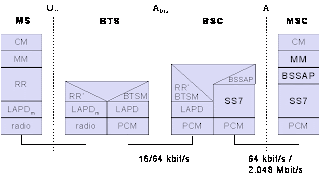
PROTOCOL ARCHITECTURE FOR SIGNALING
The main task of the physical layer comprise channel coding and error detection/correction . It uses different forward error correction (FEC) .
Physical layer contains special function such as voice activity detection(VAD),which transmits voice data only when there is a voice signal.
Signaling between entities in a GSM network requires higher layer . For this purpose the LAPDm protocol has been defined at Um interface for layer two. LAPDm has been derived from link access procedure for the D-channel in ISDN system ,which is a version of HDLC. LAPDm does not need synchronization flags or checksumming for error detection . LAPDm is also included segmentation and reassembly of data and acknowledged/ unacknowledged data transfer.
Third layer in GSM system is network layer .The lowest sublayer is the radio resource management(RR) , is implemented in the BTS and remainder is situated in the BSC .The main task of RR are setup ,maintenance ,and release of radio channels . RR also directly access the physical layer for radio information and offers a reliable connection to next higher layer. Mobility management (MM) contains functions for registration , location updating ,identification and provision of a temporary mobile
subscriber identity(TMSI) that replace the international mobile subscriber identity (IMSI) .The call management(CM) layer contains three entities : call control(CC) , short message service(SMS) , and supplementary services(SS) .SMS allows for message transfer using protocol channels SDCCH and SACCH. This layer also provides functions to send in-band tones , called dual tone multiple frequency (DTMF). Data transmission at the physical layer uses pulse code modulation (PCM) .
PCM system offer transparent 64 kbps channels, GSM allows for submultiplexing of four 16kbps channels into a single 64 kbps. The physical layer at the A interface typically includes leased lines with 2.048 Mbps capacity. LAPD is used for layer two at Abis ,BTSM for BTS management.
Signaling System No.7(SS7) is used for signaling between an MSC and a BSC. This protocol also transfer all management information between MSCs , HLR , VLRs , AuC , EIR and OMC. An MSC can also control a BSS via a BSS application part (BSSAP) .
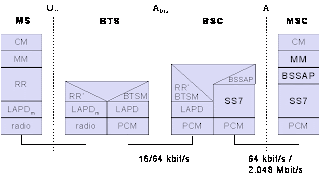
PROTOCOL ARCHITECTURE FOR SIGNALING
The main task of the physical layer comprise channel coding and error detection/correction . It uses different forward error correction (FEC) .
Physical layer contains special function such as voice activity detection(VAD),which transmits voice data only when there is a voice signal.
Signaling between entities in a GSM network requires higher layer . For this purpose the LAPDm protocol has been defined at Um interface for layer two. LAPDm has been derived from link access procedure for the D-channel in ISDN system ,which is a version of HDLC. LAPDm does not need synchronization flags or checksumming for error detection . LAPDm is also included segmentation and reassembly of data and acknowledged/ unacknowledged data transfer.
Third layer in GSM system is network layer .The lowest sublayer is the radio resource management(RR) , is implemented in the BTS and remainder is situated in the BSC .The main task of RR are setup ,maintenance ,and release of radio channels . RR also directly access the physical layer for radio information and offers a reliable connection to next higher layer. Mobility management (MM) contains functions for registration , location updating ,identification and provision of a temporary mobile
subscriber identity(TMSI) that replace the international mobile subscriber identity (IMSI) .The call management(CM) layer contains three entities : call control(CC) , short message service(SMS) , and supplementary services(SS) .SMS allows for message transfer using protocol channels SDCCH and SACCH. This layer also provides functions to send in-band tones , called dual tone multiple frequency (DTMF). Data transmission at the physical layer uses pulse code modulation (PCM) .
PCM system offer transparent 64 kbps channels, GSM allows for submultiplexing of four 16kbps channels into a single 64 kbps. The physical layer at the A interface typically includes leased lines with 2.048 Mbps capacity. LAPD is used for layer two at Abis ,BTSM for BTS management.
Signaling System No.7(SS7) is used for signaling between an MSC and a BSC. This protocol also transfer all management information between MSCs , HLR , VLRs , AuC , EIR and OMC. An MSC can also control a BSS via a BSS application part (BSSAP) .
RADIO INTERFACE
The most interesting interface in GSM system is Um interface. FDD is used for separating uplink and downlink. FDMA and TDMA is used for media access control.
GSM 900 is divided into 124 channels ,each 200khz wide using FDMA. Channel 1 and channel 124 is not used for transmission , 32 channels are reserved for organizational data and remaining 90 channels are used for voice transmission. Each 200 khz carrier is subdivided into frames that repeated continuously. The duration of a frame is 4.615 ms .A frame is subdivided into 8 GSM time slots with a duration of 577 ms .
Data is transmitted in small portions called burst .The burst is only 546.5 ms long and contains 148 bits .The remaining 30.5 ms is used as guard space to avoid overlapping with other bursts due to different path delays and give the transmitter time to turn on and off. The physical channel has a raw data rate of about 33.8kbps,each radio carrier transmitted approximately 270 kbps over Um interface .THE FIRST and last A three bits of a normal burst are all set to zero and can be used to enhance the receiver performance .the training sequence in the middle of a slot is used to adapt the parameter of the receiver to the current path propagation and select the strongest signal in case of multi path propagation. A flag S indicates where the data field contains user or network control data .A frequency correction burst allows the MS to correct the local oscillator to avoid interference with neighboring channels . synchronization burst with an extended training sequence synchronizes the MS with the BTS in time ,an access burst is used for the initial correction setup between MS and BTS an finally a dummy burst is used if no data is available for a slot .
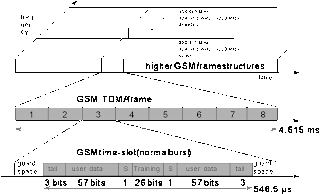
GSM TDMA FRAME SLOTS, AND BURSTS
GSM 900 is divided into 124 channels ,each 200khz wide using FDMA. Channel 1 and channel 124 is not used for transmission , 32 channels are reserved for organizational data and remaining 90 channels are used for voice transmission. Each 200 khz carrier is subdivided into frames that repeated continuously. The duration of a frame is 4.615 ms .A frame is subdivided into 8 GSM time slots with a duration of 577 ms .
Data is transmitted in small portions called burst .The burst is only 546.5 ms long and contains 148 bits .The remaining 30.5 ms is used as guard space to avoid overlapping with other bursts due to different path delays and give the transmitter time to turn on and off. The physical channel has a raw data rate of about 33.8kbps,each radio carrier transmitted approximately 270 kbps over Um interface .THE FIRST and last A three bits of a normal burst are all set to zero and can be used to enhance the receiver performance .the training sequence in the middle of a slot is used to adapt the parameter of the receiver to the current path propagation and select the strongest signal in case of multi path propagation. A flag S indicates where the data field contains user or network control data .A frequency correction burst allows the MS to correct the local oscillator to avoid interference with neighboring channels . synchronization burst with an extended training sequence synchronizes the MS with the BTS in time ,an access burst is used for the initial correction setup between MS and BTS an finally a dummy burst is used if no data is available for a slot .
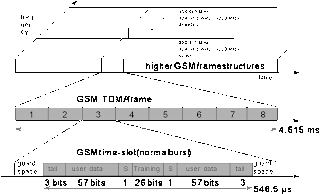
GSM TDMA FRAME SLOTS, AND BURSTS
SYSTEM ARCHITECTURE
SYSTEM ARCHITECTURE
GSM comes with hierarchical, complex system architecture containing many entities, acronyms and interfaces. GSM consists of three-sub system…
1. Radio Subsystem (RSS)
2. Network and switching subsystem(NSS)
3. Operation subsystem(OSS)
RADIO SUBSYSTEM
The RSS comprise all radio specific entities like MS and base station subsystem (BSS) .The RSS connected with NSS via A interface and NSS connected with OSS via O interface. The A interface is typically based on circuit-switched PCM-30 system carrying up to 30-64 kbit/s connection and O interface uses the Signaling System no.7 based on X.25 carrying management data to/from the RSS. The component of RSS are given below…
Base station subsystem (BSS)
A GSM network comprise many BSS, each controlled by a BSC .The BSS perform all function necessary to maintain radio connection to MS, coding/decoding of voice and rate adaptation to/ from the wireless network part. BSS contains several BTS.
¨Base transceiver station (BTS)
A BTS comprise all radio equipment i.e. antenna, signal processing, amplifiers
Necessary for the radio transmission BTS can form a radio cell or, usage sectored antenna several cells and is connected to MS via Um interface, and to the BSC via the ABIOS interface.
¨Base station controller (BSC)
The BSC basically manages the BTS .It receives radio frequencies, handle handover from one BTS to another within the BSS and performs paging of the MS.The BSC also multiplexes the radio channels onto the fixed network at the A interface.
¨ MOBILE STATION (MS)
Mobile station comprises all user needed software and hardware for the communication using gsm services.MS consists of a SUBSCRIBER IDENTITY MODULE(SIM) which stores all user data relevant to the gsm.ms can be identified with its international mobile equipment identity(IMEI) for the theft protection. Without the sim only the emergency calls are posible.SIM contains ……
1.personel identity no.(PIN)
2.PIN unblocking key(PUK).
3.Authentication key ki.
4.International mobile subscribers identity(IMSI)
The pin is used to unlock the MS. Using the wrong pin three times will lock the SIM.
The MS stores the dynamic information e.g. cipher key Kc ,temporary mobile subscriber identity (TMSI) and location area identification(LAI).It consists of three part ……
1. Mobile terminal(MT)
2. Terminal adapter(TA)
3. Terminal equipment(TE)


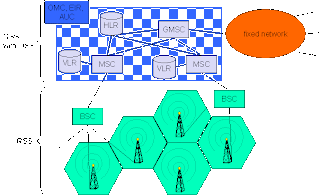

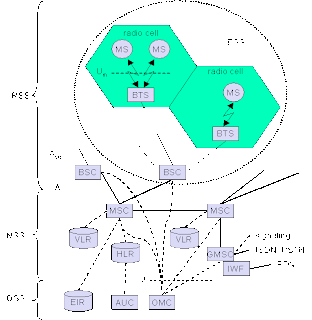

NETWORK AND SWITCHING SUBSYSTEM
The brain of GSM is formed by NSS. The NSS connects the wireless network with standard public network, performs handover between different BSS, and comprises function for worldwide location of users and supports charging, accounting, and roaming of users between different providers in different countries. The NSS consists of the following components…
Mobile services switching center (MSC): --
MSCs are high performance digital ISDN switches. They set up connections to other MSCs and to the BSCs via A interface and form the fixed backbone network of GSM system . A gateway MSC (GMSC) has additional connections to other fixed networks e.g. ISDN and PSTN. Using additional interworking functions (IWF), an MSC can also connect to public data networks (PDN) such as X.25. An MSC handles all signaling needed for connection setup, connection release and handover connections to other MSCs. The standard signaling system NO. 7(SS7) is used for this purpose.
¨HOME LOCATION REGISTER (HLR): --
The HLR is most important database in a GSM network system and store all static user relevant information like mobile subscriber ISDN number (MSISDN), subscriber services e.g. call forwarding, roaming restrictions, GPRS and inter mobile subscriber identity (IMSI). It also contains dynamic information such as current location area (LA) of the MS, the mobile subscriber roaming number (MSRN), and current VLR and MSC.
¨VISITOR LOCATION REGISTER (VLR): --
The VLR associated each MSC is a dynamic data base which stores all important information needed for the MS users currently in the la served by the MSC.If a new MS comes in to the LA the VLR is responsible to deal with it. Some VLRs in existence are capable of storing one million customers.
OPERATION SUBSYSTEM (OSS): --
It contains the necessary functions of network operation and maintenance.it contains the network entities of its own and accesses others from SS7 protocol. The following entities have been defined..
¨OPERATION AND MAINTENANCE CENTRE (OMC):
The OMC contains and monitors all other network entities via the o interface. OMCs use the telecommunication management network(TMN).
It manages traffic monitoring ,status reports of network entities, subscriber and security management or accounting and billing.
Authentication center (AuC):---
A AuC is defined to protect user identity and data transmission. The AuC contains algorithm for authentication as well as the key for encryption and generate the value needed for user authentication in the HLR.
Equipment identity register(EIR):---
The EIR is a database for all IMEIs and stores all device identification registered for this system. The EIR has a white list for valid MS and blacklist for stolen MS.A MS is useless as soon as the owner has reported a theft.
GSM comes with hierarchical, complex system architecture containing many entities, acronyms and interfaces. GSM consists of three-sub system…
1. Radio Subsystem (RSS)
2. Network and switching subsystem(NSS)
3. Operation subsystem(OSS)
RADIO SUBSYSTEM
The RSS comprise all radio specific entities like MS and base station subsystem (BSS) .The RSS connected with NSS via A interface and NSS connected with OSS via O interface. The A interface is typically based on circuit-switched PCM-30 system carrying up to 30-64 kbit/s connection and O interface uses the Signaling System no.7 based on X.25 carrying management data to/from the RSS. The component of RSS are given below…
Base station subsystem (BSS)
A GSM network comprise many BSS, each controlled by a BSC .The BSS perform all function necessary to maintain radio connection to MS, coding/decoding of voice and rate adaptation to/ from the wireless network part. BSS contains several BTS.
¨Base transceiver station (BTS)
A BTS comprise all radio equipment i.e. antenna, signal processing, amplifiers
Necessary for the radio transmission BTS can form a radio cell or, usage sectored antenna several cells and is connected to MS via Um interface, and to the BSC via the ABIOS interface.
¨Base station controller (BSC)
The BSC basically manages the BTS .It receives radio frequencies, handle handover from one BTS to another within the BSS and performs paging of the MS.The BSC also multiplexes the radio channels onto the fixed network at the A interface.
¨ MOBILE STATION (MS)
Mobile station comprises all user needed software and hardware for the communication using gsm services.MS consists of a SUBSCRIBER IDENTITY MODULE(SIM) which stores all user data relevant to the gsm.ms can be identified with its international mobile equipment identity(IMEI) for the theft protection. Without the sim only the emergency calls are posible.SIM contains ……
1.personel identity no.(PIN)
2.PIN unblocking key(PUK).
3.Authentication key ki.
4.International mobile subscribers identity(IMSI)
The pin is used to unlock the MS. Using the wrong pin three times will lock the SIM.
The MS stores the dynamic information e.g. cipher key Kc ,temporary mobile subscriber identity (TMSI) and location area identification(LAI).It consists of three part ……
1. Mobile terminal(MT)
2. Terminal adapter(TA)
3. Terminal equipment(TE)


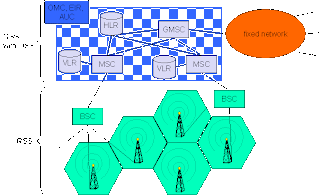

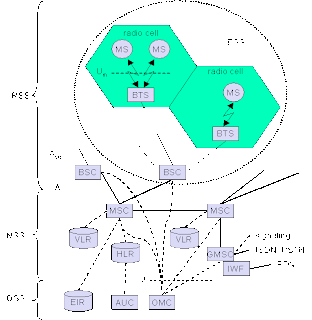

NETWORK AND SWITCHING SUBSYSTEM
The brain of GSM is formed by NSS. The NSS connects the wireless network with standard public network, performs handover between different BSS, and comprises function for worldwide location of users and supports charging, accounting, and roaming of users between different providers in different countries. The NSS consists of the following components…
Mobile services switching center (MSC): --
MSCs are high performance digital ISDN switches. They set up connections to other MSCs and to the BSCs via A interface and form the fixed backbone network of GSM system . A gateway MSC (GMSC) has additional connections to other fixed networks e.g. ISDN and PSTN. Using additional interworking functions (IWF), an MSC can also connect to public data networks (PDN) such as X.25. An MSC handles all signaling needed for connection setup, connection release and handover connections to other MSCs. The standard signaling system NO. 7(SS7) is used for this purpose.
¨HOME LOCATION REGISTER (HLR): --
The HLR is most important database in a GSM network system and store all static user relevant information like mobile subscriber ISDN number (MSISDN), subscriber services e.g. call forwarding, roaming restrictions, GPRS and inter mobile subscriber identity (IMSI). It also contains dynamic information such as current location area (LA) of the MS, the mobile subscriber roaming number (MSRN), and current VLR and MSC.
¨VISITOR LOCATION REGISTER (VLR): --
The VLR associated each MSC is a dynamic data base which stores all important information needed for the MS users currently in the la served by the MSC.If a new MS comes in to the LA the VLR is responsible to deal with it. Some VLRs in existence are capable of storing one million customers.
OPERATION SUBSYSTEM (OSS): --
It contains the necessary functions of network operation and maintenance.it contains the network entities of its own and accesses others from SS7 protocol. The following entities have been defined..
¨OPERATION AND MAINTENANCE CENTRE (OMC):
The OMC contains and monitors all other network entities via the o interface. OMCs use the telecommunication management network(TMN).
It manages traffic monitoring ,status reports of network entities, subscriber and security management or accounting and billing.
Authentication center (AuC):---
A AuC is defined to protect user identity and data transmission. The AuC contains algorithm for authentication as well as the key for encryption and generate the value needed for user authentication in the HLR.
Equipment identity register(EIR):---
The EIR is a database for all IMEIs and stores all device identification registered for this system. The EIR has a white list for valid MS and blacklist for stolen MS.A MS is useless as soon as the owner has reported a theft.
Gloabl System For Mobile Communication
1. INTRODUCTION
2. MOBILE SERVICES
3. SYSTEM ARCHITECTURE
4. RADIO INTERFACE
5. LOCALIZATION AND CALLING
6. HANDOVER
7. SECURITY
8. NEW DATA SERVICES
INTRODUCTION
As the wired communication was facing many problems and was thought to be costly. Therefore it was desired by the users as well as by the service providers that the wireless communication must be brought in to use in order to provides some additional facilities to its users with lesser cost and difficulty. The complete mobile communication is divided into three generations…. …
1. First Generation(1980-1990)
2. Second Generation(1991-2005)
3. Third Generation(2002-onward)
In the first generation we were using analog modulation. It was completely based on analog communication. The important technology were given below
1. AMPS (824 –849mhz (uplink) 869-894mhz(down link))
2. TACS (890 –905mhz (uplink) 935-960mhz(down link))
3. NMT (890 –905mhz (uplink) 935-960mhz(down link))
NTT(860 –885mhz (uplink) 915-940mhz(down link))
Second generation of mobile communication is based on digital communication. It is mostly divided into two part GSM and CDMA.
GSM stands for Global System for Mobile Communication.
GSM is the most popular digital system with approximately 70% market share. GSM is the most successful digital system of second generation in the world today. In past it was known as GROUP SPECIAL MOBILE (GSM). GSM is initially deployed in EUROPE using 890-915mhz for uplink and 935-960 MHz for downlink this system is also called GSM 900. GSM 1800(1710 –1785mhz (uplink) 1805-1880mhz(down link)) also called DCS (digital cellular system). GSM 1900(1850 –1910mhz (uplink) 1930-1990mhz(down link)) also called PCS (personal communication system). A GSM system that has been introduced in several European countries for rail-board is GSM –rail. Today many providers all over the world use GSM (more than 184 countries in Asia, Africa, Europe, Australia, and America)
GSM has defined three different type of services………
1. BEARER SERVICESS
2. TELE SERVICESS
3. SUPPLEMENTRY SERVICESS
A mobile station MS is connected to the GSM public land mobile network (PLMN) via the Um interface .This network is connected to transit network ISDN or PSTN.
1.BEARER SERVICESS
Bearer services permit transparent and non-transparent ,synchronous or asynchronous data transmission. Transparent bearer services only use the function of the physical layer. Data transmission has a constant delay throughput if no transmission errors occur. The only mechanism to increase the Transmission quality is the use of forward error correction (FEC).
Non- Transparent bearer services use protocols of layers two and three to implement error correction and flow control. The services use the transparent bearer services, adding radio link protocol (RLP). This protocol comprises mechanism of high-level data link protocol (HDLC).
· data service (circuit switched)
· synchronous: 2.4, 4.8 or 9.6 kbit/s
· asynchronous: 300 - 1200 bit/s
· data service (packet switched)
· synchronous: 2.4, 4.8 or 9.6 kbit/s
· asynchronous: 300 – 9600 bit/s
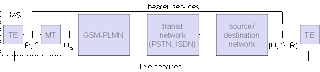
TELE SERVICES
GSM mainly focuses on voice-oriented Tele services. These comprise encrypted voice transmission, message services, and basic data communication with terminals known as from PSTN or ISDN. The primary goal of GSM was the provision of high-quality digital voice transmission, offering at least the typical bandwidth of 3.1khz of analog phone. Another services offered by GSM are the emergency number. A useful service offered by GSM is short message service (SMS) with transmission upto 160 characters. SMS message do not use the standard data channels of GSM but exploit unused capacity in the signaling channels. The successor of SMS is EMS with capacity upto 760 characters and used for transmission of animated picture, images ring tones. Multimedia message services (MMS) is available for transmission of large picture, short video clips. Another non-voice available service is group 3 fax.
SUPPLEMENTARY SERVICES
GSM can offer supplementary services like identification, call redirection or forwarding of ongoing call. Standard ISDN feature like closed user group and multiparty communication may be available.
· identification: forwarding of caller number
· suppression of number forwarding
· automatic call-back
· conferencing with up to 7 participants
· Locking of the mobile terminal (incoming or outgoing calls
2. MOBILE SERVICES
3. SYSTEM ARCHITECTURE
4. RADIO INTERFACE
5. LOCALIZATION AND CALLING
6. HANDOVER
7. SECURITY
8. NEW DATA SERVICES
INTRODUCTION
As the wired communication was facing many problems and was thought to be costly. Therefore it was desired by the users as well as by the service providers that the wireless communication must be brought in to use in order to provides some additional facilities to its users with lesser cost and difficulty. The complete mobile communication is divided into three generations…. …
1. First Generation(1980-1990)
2. Second Generation(1991-2005)
3. Third Generation(2002-onward)
In the first generation we were using analog modulation. It was completely based on analog communication. The important technology were given below
1. AMPS (824 –849mhz (uplink) 869-894mhz(down link))
2. TACS (890 –905mhz (uplink) 935-960mhz(down link))
3. NMT (890 –905mhz (uplink) 935-960mhz(down link))
NTT(860 –885mhz (uplink) 915-940mhz(down link))
Second generation of mobile communication is based on digital communication. It is mostly divided into two part GSM and CDMA.
GSM stands for Global System for Mobile Communication.
GSM is the most popular digital system with approximately 70% market share. GSM is the most successful digital system of second generation in the world today. In past it was known as GROUP SPECIAL MOBILE (GSM). GSM is initially deployed in EUROPE using 890-915mhz for uplink and 935-960 MHz for downlink this system is also called GSM 900. GSM 1800(1710 –1785mhz (uplink) 1805-1880mhz(down link)) also called DCS (digital cellular system). GSM 1900(1850 –1910mhz (uplink) 1930-1990mhz(down link)) also called PCS (personal communication system). A GSM system that has been introduced in several European countries for rail-board is GSM –rail. Today many providers all over the world use GSM (more than 184 countries in Asia, Africa, Europe, Australia, and America)
GSM has defined three different type of services………
1. BEARER SERVICESS
2. TELE SERVICESS
3. SUPPLEMENTRY SERVICESS
A mobile station MS is connected to the GSM public land mobile network (PLMN) via the Um interface .This network is connected to transit network ISDN or PSTN.
1.BEARER SERVICESS
Bearer services permit transparent and non-transparent ,synchronous or asynchronous data transmission. Transparent bearer services only use the function of the physical layer. Data transmission has a constant delay throughput if no transmission errors occur. The only mechanism to increase the Transmission quality is the use of forward error correction (FEC).
Non- Transparent bearer services use protocols of layers two and three to implement error correction and flow control. The services use the transparent bearer services, adding radio link protocol (RLP). This protocol comprises mechanism of high-level data link protocol (HDLC).
· data service (circuit switched)
· synchronous: 2.4, 4.8 or 9.6 kbit/s
· asynchronous: 300 - 1200 bit/s
· data service (packet switched)
· synchronous: 2.4, 4.8 or 9.6 kbit/s
· asynchronous: 300 – 9600 bit/s
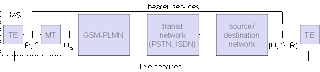
TELE SERVICES
GSM mainly focuses on voice-oriented Tele services. These comprise encrypted voice transmission, message services, and basic data communication with terminals known as from PSTN or ISDN. The primary goal of GSM was the provision of high-quality digital voice transmission, offering at least the typical bandwidth of 3.1khz of analog phone. Another services offered by GSM are the emergency number. A useful service offered by GSM is short message service (SMS) with transmission upto 160 characters. SMS message do not use the standard data channels of GSM but exploit unused capacity in the signaling channels. The successor of SMS is EMS with capacity upto 760 characters and used for transmission of animated picture, images ring tones. Multimedia message services (MMS) is available for transmission of large picture, short video clips. Another non-voice available service is group 3 fax.
SUPPLEMENTARY SERVICES
GSM can offer supplementary services like identification, call redirection or forwarding of ongoing call. Standard ISDN feature like closed user group and multiparty communication may be available.
· identification: forwarding of caller number
· suppression of number forwarding
· automatic call-back
· conferencing with up to 7 participants
· Locking of the mobile terminal (incoming or outgoing calls
Changing Connection String of DataEnvironment at runtime
Changing Connection String of DataEnvironment at runtime
If DataEnvironment1.Connection1.State = adStateOpen Then
DataEnvironment1.Connection1.Close
Else
DataEnvironment1.Connection1.ConnectionString = strConnString
DataEnvironment1.Connection1.Open
End If
strConnString= Data Provider=SQLOLEDB;Data Source=ServerAddress;Initial Catalog=DataBase;User ID=Username;Password=Password;
it will work when u r using single command but when u will use child command . it will show error to solve it u have change ur connection string by adding Data Shape as given below. Data Shape is used for relational database in report design.
strConnString= Provider=MSDataShape;Data Provider=SQLOLEDB;Data Source=erverAddress;Initial Catalog=DataBase;User ID=Username;Password=Password;
If DataEnvironment1.Connection1.State = adStateOpen Then
DataEnvironment1.Connection1.Close
Else
DataEnvironment1.Connection1.ConnectionString = strConnString
DataEnvironment1.Connection1.Open
End If
strConnString= Data Provider=SQLOLEDB;Data Source=ServerAddress;Initial Catalog=DataBase;User ID=Username;Password=Password;
it will work when u r using single command but when u will use child command . it will show error to solve it u have change ur connection string by adding Data Shape as given below. Data Shape is used for relational database in report design.
strConnString= Provider=MSDataShape;Data Provider=SQLOLEDB;Data Source=erverAddress;Initial Catalog=DataBase;User ID=Username;Password=Password;
Saturday, November 17, 2007
Messanger /Common Chatroom in asp.net
Hi thsi is simple Messanger application in asp.net.
for this u need to create two derault.aspx and default2.aspx two pages and one global.aspx file.
u can add some more feature but it is very simple use of application variable.
Dfault.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Collections;
public partial class _Default : System.Web.UI.Page
{
Hashtable schedule = new Hashtable();
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
int i = 0;
for (i = data.Length - 1; i > 0; i--)
{
if (data[i] == "")
break;
data[i] = data[i - 1];
}
data[i] = "<strong> Welcome " + TextBox1.Text + " :</strong> ";
Session["name"] = "<strong> "+
TextBox1.Text + "</strong> ";
Server.Transfer("Default2.aspx");
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title> Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:TextBox ID="TextBox1" runat="server" Style="z-index: 103; left: 398px; position: absolute;
top: 152px"> </asp:TextBox>
<asp:Label ID="Label1" runat="server" Font-Bold="True" ForeColor="#00C000" Style="z-index: 105;
left: 273px; position: absolute; top: 155px" Text=" Nick Name"> </asp:Label>
<asp:Button ID="Button1" runat="server" Style="z-index: 101;
left: 455px; position: absolute; top: 193px" Text="enter" OnClick="Button1_Click" />
</div>
</form>
</body>
</html>
Global.aspx
<%@ Application Language="C#" %>
<script runat="server">
void Application_Start(object sender, EventArgs e)
{
Application["msg"]= new string[20];
}
void Application_End(object sender, EventArgs e)
{
// Code that runs on application shutdown
}
void Application_Error(object sender, EventArgs e)
{
// Code that runs when an unhandled error occurs
}
void Session_Start(object sender, EventArgs e)
{
string name = "";
}
void Session_End(object sender, EventArgs e)
{
// Code that runs when a session ends.
// Note: The Session_End event is raised only when the sessionstate mode
// is set to InProc in the Web.config file. If session mode is set to StateServer
// or SQLServer, the event is not raised.
}
</script>
Default2.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Xml.Xsl;
using System.Xml.XPath;
public partial class Default2 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
for (int i = 0; i <data.Length; i++)
Response.Write(data[i]+"<br> ");
Response.AppendHeader("Refresh", "60; URL=http://localhost:3106/TestAsp/Default2.aspx");
}
protected void Button1_Click(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
int i = 0;
for ( i= data.Length - 1; i > 0; i--)
{
if (data[i] == "")
break;
data[i] = data[i - 1];
}
data[i] = (string)Session["name"] + TextBox1.Text;
Application["msg"] = data;
}
}
Default2.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title> Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Style="z-index: 100;
left: 147px; position: absolute; top: 522px" Text="Submit" />
<asp:TextBox ID="TextBox1" runat="server" Style="z-index: 101; left: 133px; position: absolute;
top: 483px" OnTextChanged="TextBox1_TextChanged" Width="362px"> </asp:TextBox>
<asp:Label ID="Label1" runat="server" Font-Bold="True" ForeColor="#00C000" Style="z-index: 103;
left: 21px; position: absolute; top: 487px" Text="Write Message" Width="102px"> </asp:Label>
</div>
</form>
</body>
</html>
for this u need to create two derault.aspx and default2.aspx two pages and one global.aspx file.
u can add some more feature but it is very simple use of application variable.
Dfault.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Collections;
public partial class _Default : System.Web.UI.Page
{
Hashtable schedule = new Hashtable();
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
int i = 0;
for (i = data.Length - 1; i > 0; i--)
{
if (data[i] == "")
break;
data[i] = data[i - 1];
}
data[i] = "<strong> Welcome " + TextBox1.Text + " :</strong> ";
Session["name"] = "<strong> "+
TextBox1.Text + "</strong> ";
Server.Transfer("Default2.aspx");
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title> Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:TextBox ID="TextBox1" runat="server" Style="z-index: 103; left: 398px; position: absolute;
top: 152px"> </asp:TextBox>
<asp:Label ID="Label1" runat="server" Font-Bold="True" ForeColor="#00C000" Style="z-index: 105;
left: 273px; position: absolute; top: 155px" Text=" Nick Name"> </asp:Label>
<asp:Button ID="Button1" runat="server" Style="z-index: 101;
left: 455px; position: absolute; top: 193px" Text="enter" OnClick="Button1_Click" />
</div>
</form>
</body>
</html>
Global.aspx
<%@ Application Language="C#" %>
<script runat="server">
void Application_Start(object sender, EventArgs e)
{
Application["msg"]= new string[20];
}
void Application_End(object sender, EventArgs e)
{
// Code that runs on application shutdown
}
void Application_Error(object sender, EventArgs e)
{
// Code that runs when an unhandled error occurs
}
void Session_Start(object sender, EventArgs e)
{
string name = "";
}
void Session_End(object sender, EventArgs e)
{
// Code that runs when a session ends.
// Note: The Session_End event is raised only when the sessionstate mode
// is set to InProc in the Web.config file. If session mode is set to StateServer
// or SQLServer, the event is not raised.
}
</script>
Default2.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Xml.Xsl;
using System.Xml.XPath;
public partial class Default2 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
for (int i = 0; i <data.Length; i++)
Response.Write(data[i]+"<br> ");
Response.AppendHeader("Refresh", "60; URL=http://localhost:3106/TestAsp/Default2.aspx");
}
protected void Button1_Click(object sender, EventArgs e)
{
string[] data = (string[])Application["msg"];
int i = 0;
for ( i= data.Length - 1; i > 0; i--)
{
if (data[i] == "")
break;
data[i] = data[i - 1];
}
data[i] = (string)Session["name"] + TextBox1.Text;
Application["msg"] = data;
}
}
Default2.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title> Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Style="z-index: 100;
left: 147px; position: absolute; top: 522px" Text="Submit" />
<asp:TextBox ID="TextBox1" runat="server" Style="z-index: 101; left: 133px; position: absolute;
top: 483px" OnTextChanged="TextBox1_TextChanged" Width="362px"> </asp:TextBox>
<asp:Label ID="Label1" runat="server" Font-Bold="True" ForeColor="#00C000" Style="z-index: 103;
left: 21px; position: absolute; top: 487px" Text="Write Message" Width="102px"> </asp:Label>
</div>
</form>
</body>
</html>
Friday, November 16, 2007
Sending mail Thorough c#.Net
Sending mail Thorough c#.Net
For it to work U have to have a smtp server and credential for that server.
If u have gamilid u can also use it.
using System;
using System.Collections.Generic;
using System.Text;
using System.Net.Mail;
using System.Net.Mime;
using System.Configuration;
namespace mail
{
class Program
{
static void Main(string[] args)
{
MailMessage msg = new MailMessage();
msg.From = new MailAddress("abc@gmail.com", "Hi..........");
msg.To.Add(“aa@abc.com”);
msg.Subject = "Sending Mail Through C#";
msg.Body = " This is first mail through C#.net For this u have to gmail id .there are many other server which u can use but gmail is better one. Or u have ur own Smtp seever. ";
SmtpClient client = new SmtpClient();
client.Credentials =
new System.Net.NetworkCredential("abc@gmail.com", "password");
// u must provide login id and password.
client.Port = 587;//or use 587
msg.Priority = MailPriority.High;
msg.IsBodyHtml = true;
client.Host = "smtp.gmail.com";
client.EnableSsl = true;
client.Send(msg);
}
}
Hiding URL of page in Asp.Net
Hiding URL of page in Asp.Net
Suppose u have main pages default.aspx. and u are using query string to tranfer sensitive data. And u want to hide it.Now ur next url are like
Localhost:1528//Abc/product.asp?a=100;
Localhost:1528//Abc/product.asp?a=50;
Localhost:1528//Abc/product.asp?a=70;
Now u want instead of showing these url a coomon url as
Localhost:1528//Abc/allproduct.aspx
For this go to allproduct.aspx from default.aspx and in
Page_load method use Serve.Transfer(Localhost:1528//Abc/product.asp?a=50);
Now ur getting the information from this page Localhost:1528//Abc/product.asp?a=50 but ur url will showing as Localhost:1528//Abc/allproduct.aspx
So when ur tranfering from default.aspx to allprpduct.aspx just transfer the appropriate url eg Localhost:1528//Abc/ product.asp?a=50
Thursday, November 1, 2007
Progressbar in console application
This is a program for progess bar in Console application.
We specially constrolling the postion of cursor .
plz don use Console.Writeline();
because it will go to next line.
using System;
namespace testcsharp
{
class Program
{
static void Main(string[] args)
{
ConsoleLineProgressBar (10, 100);
ConsoleLineProgressBar (20, 100);
ConsoleLineProgressBar (30, 100);
ConsoleLineProgressBar (40, 100);
ConsoleLineProgressBar (70, 100);
ConsoleLineProgressBar (100, 100);
}
private static void ConsoleLineProgressBar(int progress, int total)
{
Console.CursorLeft = 0;
Console.Write("[");
Console.CursorLeft = 42;
Console.Write("]");
Console.CursorLeft = 1;
float onechunk = 40.0f / total;
int position = 1;
for (int i = 0; i < onechunk * progress; i++)
{
Console.BackgroundColor = ConsoleColor.Red;
Console.CursorLeft = position++;
Console.Write(" ");
}
for (int i = position; i < 41; i++)
{
Console.BackgroundColor = ConsoleColor.Black;
Console.CursorLeft = position++;
Console.Write(" ");
}
Console.CursorLeft = 45;
Console.BackgroundColor = ConsoleColor.Black;
Console.ForegroundColor = ConsoleColor.Green;
Console.Write(progress.ToString() + " Record has been Processed ");
}
}
}
We specially constrolling the postion of cursor .
plz don use Console.Writeline();
because it will go to next line.
using System;
namespace testcsharp
{
class Program
{
static void Main(string[] args)
{
ConsoleLineProgressBar (10, 100);
ConsoleLineProgressBar (20, 100);
ConsoleLineProgressBar (30, 100);
ConsoleLineProgressBar (40, 100);
ConsoleLineProgressBar (70, 100);
ConsoleLineProgressBar (100, 100);
}
private static void ConsoleLineProgressBar(int progress, int total)
{
Console.CursorLeft = 0;
Console.Write("[");
Console.CursorLeft = 42;
Console.Write("]");
Console.CursorLeft = 1;
float onechunk = 40.0f / total;
int position = 1;
for (int i = 0; i < onechunk * progress; i++)
{
Console.BackgroundColor = ConsoleColor.Red;
Console.CursorLeft = position++;
Console.Write(" ");
}
for (int i = position; i < 41; i++)
{
Console.BackgroundColor = ConsoleColor.Black;
Console.CursorLeft = position++;
Console.Write(" ");
}
Console.CursorLeft = 45;
Console.BackgroundColor = ConsoleColor.Black;
Console.ForegroundColor = ConsoleColor.Green;
Console.Write(progress.ToString() + " Record has been Processed ");
}
}
}
Excel Report in .Net 2.0 Using C#
This a program to gererate Excel report through .net using c#
Steps
1. u need to add areference to com component microsoft.Office.Excel dll
2. Store the result data from table to a data table loop it to generate the report.
add reference as using Excel;
using System;
using System.Collections.Generic;
using System.Text;
using System.Collections;
using System.IO;
using System.Data.SqlClient;
using System.Data;
using Excel;
namespace Student
{
class Program
{
static void Main(string[] args)
{
Excel.Application oXL = new Excel.Application();
Excel.Workbook oBook;
Excel.Worksheet oSheet, oSheet1, oSheet2;
string fileNameMerchant = null;
SqlConnection sCon=null;
try
{
// Initialization of Database connections
sCon = new SqlConnection(@"Data Source=.\SqlExpress; Initial catalog=aspnetdb; integrated security =true");
sCon.Open();
oBook = oXL.Workbooks.Add(Type.Missing);
oXL.Visible = false;
if (oBook.Worksheets.Count > 0)
{
oSheet = (Excel.Worksheet)oBook.Sheets[1];
oSheet.Activate();
SqlDataAdapter da = new SqlDataAdapter("select *from student", sCon);
DataSet ds = new DataSet();
da.Fill(ds);
Range rg = oSheet.get_Range("A1", "B1");
rg.Select();
rg = oSheet.get_Range(“A1”, Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = " Student Detail ";
int 3 = 1;
string s = "A" + x.ToString();
rg = oSheet.get_Range(s.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = "First name";
s = "B" + x.ToString();
rg = oSheet.get_Range(s.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = "Last name";
int i = 0;
x++;
int count = ds.Tables[0].Rows.Count;
while (i < count)
{
string ss = "A" + x.ToString();
rg = oSheet.get_Range(ss.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 40;
rg.HorizontalAlignment = Excel.Constants.xlRight;
rg.Value2 = "'" + ds.Tables[0].Rows[i][0].ToString();
ss = "B" + x.ToString();
rg = oSheet.get_Range(ss.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 40;
rg.HorizontalAlignment = Excel.Constants.xlRight;
rg.Value2 = ds.Tables[0].Rows[i][1];
x++;
i++;
}
}
fileNameMerchant = System.Environment.CurrentDirectory+"/"+"Studentdetail.xls";
oXL.ActiveWorkbook.SaveAs(fileNameMerchant, Excel.XlFileFormat.xlWorkbookNormal, Type.Missing, Type.Missing,
Type.Missing, Type.Missing, Excel.XlSaveAsAccessMode.xlNoChange,
Type.Missing, Type.Missing, Type.Missing, Type.Missing, Type.Missing);
oXL.ActiveWorkbook.Close(true, fileNameMerchant, false);
Console.WriteLine("Excel Repor has ben generated successfully at " + fileNameMerchant);
Console.WriteLine("Press any key to close the application");
Console.Read();
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
finally
{
sCon.Close();
}
}
}
}
Steps
1. u need to add areference to com component microsoft.Office.Excel dll
2. Store the result data from table to a data table loop it to generate the report.
add reference as using Excel;
using System;
using System.Collections.Generic;
using System.Text;
using System.Collections;
using System.IO;
using System.Data.SqlClient;
using System.Data;
using Excel;
namespace Student
{
class Program
{
static void Main(string[] args)
{
Excel.Application oXL = new Excel.Application();
Excel.Workbook oBook;
Excel.Worksheet oSheet, oSheet1, oSheet2;
string fileNameMerchant = null;
SqlConnection sCon=null;
try
{
// Initialization of Database connections
sCon = new SqlConnection(@"Data Source=.\SqlExpress; Initial catalog=aspnetdb; integrated security =true");
sCon.Open();
oBook = oXL.Workbooks.Add(Type.Missing);
oXL.Visible = false;
if (oBook.Worksheets.Count > 0)
{
oSheet = (Excel.Worksheet)oBook.Sheets[1];
oSheet.Activate();
SqlDataAdapter da = new SqlDataAdapter("select *from student", sCon);
DataSet ds = new DataSet();
da.Fill(ds);
Range rg = oSheet.get_Range("A1", "B1");
rg.Select();
rg = oSheet.get_Range(“A1”, Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = " Student Detail ";
int 3 = 1;
string s = "A" + x.ToString();
rg = oSheet.get_Range(s.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = "First name";
s = "B" + x.ToString();
rg = oSheet.get_Range(s.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 30;
rg.Font.Bold = true;
rg.Font.Name = "Arial";
rg.Font.Size = 10;
rg.Value2 = "Last name";
int i = 0;
x++;
int count = ds.Tables[0].Rows.Count;
while (i < count)
{
string ss = "A" + x.ToString();
rg = oSheet.get_Range(ss.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 40;
rg.HorizontalAlignment = Excel.Constants.xlRight;
rg.Value2 = "'" + ds.Tables[0].Rows[i][0].ToString();
ss = "B" + x.ToString();
rg = oSheet.get_Range(ss.ToString(), Type.Missing);
rg.Cells.ColumnWidth = 40;
rg.HorizontalAlignment = Excel.Constants.xlRight;
rg.Value2 = ds.Tables[0].Rows[i][1];
x++;
i++;
}
}
fileNameMerchant = System.Environment.CurrentDirectory+"/"+"Studentdetail.xls";
oXL.ActiveWorkbook.SaveAs(fileNameMerchant, Excel.XlFileFormat.xlWorkbookNormal, Type.Missing, Type.Missing,
Type.Missing, Type.Missing, Excel.XlSaveAsAccessMode.xlNoChange,
Type.Missing, Type.Missing, Type.Missing, Type.Missing, Type.Missing);
oXL.ActiveWorkbook.Close(true, fileNameMerchant, false);
Console.WriteLine("Excel Repor has ben generated successfully at " + fileNameMerchant);
Console.WriteLine("Press any key to close the application");
Console.Read();
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
finally
{
sCon.Close();
}
}
}
}
SilverLight 1.1
SilverLight
It was in the past when internet is used only for sharing information. At that time we were only using simple static pages and web based application are based on HTML. Then thing had been changed and it was time for dynamic pages and technology as JSP, Asp then ASP.net. Till asp.net things has been drastically changed. Now u can not seen even single static page. Then we have acrobat flash player and we have now much interactive and media specific pages. See the every popular web sites and you will find the Font pages with some video or flash presentation. This one field where asp.net lacking. As Microsoft want to dominate the market, it’s only not want but it does. And come up SilverLight . So Silverlight is Microsoft technology for making rich web based application much more media rich and eye catching.
SilverLight 1.0
For making application u need to have install silverligh 1.0 http://silverlight.net
For making silverlight UI interface defice it in .xmal file. And refernce it from ur .htm file. Simple steps are as
Hi.xaml as
It was in the past when internet is used only for sharing information. At that time we were only using simple static pages and web based application are based on HTML. Then thing had been changed and it was time for dynamic pages and technology as JSP, Asp then ASP.net. Till asp.net things has been drastically changed. Now u can not seen even single static page. Then we have acrobat flash player and we have now much interactive and media specific pages. See the every popular web sites and you will find the Font pages with some video or flash presentation. This one field where asp.net lacking. As Microsoft want to dominate the market, it’s only not want but it does. And come up SilverLight . So Silverlight is Microsoft technology for making rich web based application much more media rich and eye catching.
SilverLight 1.0
For making application u need to have install silverligh 1.0 http://silverlight.net
For making silverlight UI interface defice it in .xmal file. And refernce it from ur .htm file. Simple steps are as
Hi.xaml as
<Canvas xmlns="http://schemas.microsoft.com/client/2007">
<Ellipse Width="80" Height="80">
<Ellipse.Fill>
<LinearGradientBrush>
<GradientStop Offset="0" Color="Red"/>
<GradientStop Offset="0.2" Color="Orange"/>
<GradientStop Offset="0.4" Color="Yellow"/>
<GradientStop Offset="0.6" Color="Green"/>
<GradientStop Offset="0.8" Color="Blue"/>
<GradientStop Offset="1" Color="Purple"/>
</LinearGradientBrush>
</Ellipse.Fill>
</Ellipse>
<Ellipse Canvas.Left="25" Canvas.Top="50" Width="100" Height="100" StrokeThickness="10">
<Ellipse.Fill>
<SolidColorBrush Color="Red"/>
</Ellipse.Fill>
<Ellipse.Stroke>
<LinearGradientBrush>
<GradientStop Offset="0" Color="Red"/>
<GradientStop Offset="0.2" Color="Orange"/>
<GradientStop Offset="0.4" Color="Yellow"/>
<GradientStop Offset="0.6" Color="Green"/>
<GradientStop Offset="0.8" Color="Blue"/>
<GradientStop Offset="1" Color="Purple"/>
</LinearGradientBrush>
</Ellipse.Stroke>
</Ellipse>
</Convas>
xmlns="http://schemas.microsoft.com/client/2007
Note : it compulsory and u need to define it in Convas element as attribute.
XAML
its Extensible application markup language . it is xml based and used to define silverlight UI.
Now am hi.htm file as
<html>
<head>
<title>SilverLight</title>
</head>
<body>
<object type="application/x-silverlight" id="silver1" width="500" height="500">
<param name="source" value="hi.xaml"/>
<param name="background" value="Yellow"/>
</object>
</body>
</html>
Now in .htm file u need reference the .xaml file . Here we are using object to refer the .xaml file . if u are using internet explorer then use object otherwise use embed Object as
<embed type="application/x-silverlight" id="silver1" width="500" height="500">
source ="hi.xaml background ="Yellow"/>
</textarea >
there is not much more difference in object and embed .better to use embed for general pupuse.
If You want do all these work by silverlight.js and createSilverlight.js. then it will be more sophisticated and u can handle many more event using this createSilverlight.js,
Silverlight,js
it a javascript file provided by Microsoft u cant not make nay change in it.
createSilverlight.js
This JavaScript file contains one parameterless method, createSilverlight. This method is a template within which you call the createObject or createObjectEx method, both of which are defined by Silverlight.js. Specific parameters to createObject (or packaged parameters to createObjectEx) specify the Silverlight plugin's size on the HTML page and reference the XAML markup file that contains the Silverlight UI definition. Because the method parameters change per application (for instance the name of the source XAML file), each Silverlight-based application typically has a unique CreateSilverlight.js filecreateSilverlight.js looks like this
function createSilverlight()
{
Silverlight.createObjectEx(
{
source: "hi.xaml",
parentElement: document.body,
id: "silverlightControl",
properties: { width: "100%", height: "100%", version: "1.0" },
events: { onLoad: onLoad, onError: onSilverlightError }
}
)
}
Now u need to refer both silverlight.js and createsilver.js from your .htm file like
Hi.htm looks as
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Silverlight 1.0 Unleashed: Animating on MouseEnter and MouseLeave</title>
<script type="text/javascript" src="Silverlight.js"></script>
<script type="text/javascript" src="CreateSilverlight.js"></script>
<style type="text/css">body { margin: 0px }</style>
</head>
<body>
<script type="text/javascript">createSilverlight();</script>
</body>
</html>
This all about Silverlight.1.0 but presently we have Silverlight 1.1
SilverLight 1.1
Its more sofiticated and rich than silverlight 1.0. it a sub part of .net 3.0 framework. Now you can use any compiled .ddl file in .xmal file . You can use code behind file for handling some more event and defining some types which is not part of xmal. Then you can use your own definec=d types from xmal file to make application more web rich.
In Visual Studio 2008 default silver light application looks like this
It contain the following file.
.htm: Typically, this will be named default.html or something similar. The Visual Studio template uses the file name TestPage.html. This is the file that provides the basic entry point to a browser. It incorporates the basic Silverlight browser control and includes at least two JavaScript file references through SCRIPT tags with SRC values for CreateSilverlight.js and Silverlight.js (see the following descriptions of these files). The root HTML file can also contain other HTML content that displays around the Silverlight content.
CreateSilverlight.js: In the Visual Studio templates, this file is named TestPage.html.js. This JavaScript file contains one parameterless method, createSilverlight. This method is a template within which you call the createObject or createObjectEx method, both of which are defined by Silverlight.js. Specific parameters to createObject (or packaged parameters to createObjectEx) specify the Silverlight plugin's size on the HTML page and reference the XAML markup file that contains the Silverlight UI definition. Because the method parameters change per application (for instance the name of the source XAML file), each Silverlight-based application typically has a unique CreateSilverlight.js file.
Silverlight.js: This JavaScript file defines the createObject and createObjectEx methods that you call from CreateSilverlight.js to instantiate the Silverlight control in the HTML page. It also provides code to handle the client user experience if the Silverlight control is not present. You host this file on your site, and per the License Agreement you cannot modify its contents (review the License Agreement in the Silverlight SDK for details). If you write your own JavaScript code for your application, you must add this code to a different script file.
Page.xaml: This XAML file is referenced as the "source" parameter by your createSilverlight or createSilverlightEx method call. It defines the UI that will appear as the Silverlight content. The root element tag includes the minimum xmlns definitions that are required for a working Silverlight-based application. Canvas is the typical root element because it provides the widest support for UI composition. If your Silverlight-based application uses managed code for event handling, the root element includes an x:Class attribute, which enables you to reference handlers that are defined in your managed code from XAML.
If your application uses managed code, your Silverlight project also includes the following files:
Page.xaml.cs (or .vb): This file compiles into the assembly that provides the managed code event handlers at run time. The code should define the same class that was specified in the x:Class value in Page.xaml. Also, project build settings must build the assembly using the name that is specified in the URI portion of the x:Class value. You write your event handlers as members of this class. Typically, you also place this class in a namespace, and that namespace is in the managed code definition and included in the x:Class definition in Page.xaml. For Visual Basic, the namespace is the default namespace as specified in project settings. The managed code source file should not be deployed; only the output assembly is necessary for your deployed application.
If you are writing a more sophisticated application, you can use multiple code files to create the assembly, but one of these files must define the class referenced in the XAML
After compling application u will get a appliactionname.dll file in your /ClientBin folder. Which will be used by xmal file.
This is all about SilverLight 1.1
Subscribe to:
Posts (Atom)